1. How To Install JDK on Windows
Step 0: Un-Install Older Version(s) of JDK/JRE
I recommend that you install only the latest JDK. Although you can install multiple versions of JDK/JRE concurrently, it is messy.
If you have previously installed older version(s) of JDK/JRE, un-install ALL of them. Goto “Control Panel” ⇒ (optional) “Programs” ⇒ “Programs and Features” ⇒ Un-install ALL programs begin with “Java”, such as “Java SE Development Kit …”, “Java SE Runtime …”, “Java X Update …”, and etc.
Step 1: Download JDK
- Goto Java SE download site @ http://www.oracle.com/technetwork/java/javase/downloads/index.html.
- Under “Java Platform, Standard Edition” ⇒ “Java SE 13.0.{x}”, where {x} denotes a fast running security-update number ⇒ Click the “Oracle JDK Download” button.
- Under “Java SE Development Kit 13.0.{x}” ⇒ Check “Accept License Agreement”.
- Choose the JDK for your operating system, i.e., “Windows”. Download the “exe” installer (e.g., “
jdk-13.0.{x}_windows-x64_bin.exe
” – about 159MB).
Step 2: Install JDK
Run the downloaded installer (e.g., “jdk-13.0.{x}_windows-x64_bin.exe
“), which installs both the JDK and JRE.
By default, JDK is installed in directory “C:\Program Files\Java\jdk-13.0.{x}
“, where {x}
denotes the update number. Accept the defaults and follow the screen instructions to install JDK.
Use your “File Explorer”, navigate to "C:\Program Files\Java"
to inspect the sub-directories. Take note of your JDK installed directory jdk-13.0.{x}
, in particular, the update number {x}
, which you will need in the next step.
I shall refer to the JDK installed directory as <JAVA_HOME>
, hereafter, in this article.
Step 3: Include JDK’s “bin” Directory in the PATH
Windows’ Command Prompt (CMD
) searches the current directory and the directories listed in the PATH
environment variable (or system variable) for executable programs. JDK’s programs (such as Java compiler "javac.exe
” and Java runtime "java.exe
“) reside in the sub-directory “bin
” of the JDK installed directory. You need to include JDK’s “bin
” in the PATH
to run the JDK programs.
To edit the PATH
environment variable in Windows 10:
- Launch “Control Panel” ⇒ (Optional) “System and Security” ⇒ “System” ⇒ Click “Advanced system settings” on the left pane.
- Switch to “Advanced” tab ⇒ Click “Environment Variables” button.
- Under “System Variables” (the bottom pane), scroll down to select variable “Path” ⇒ Click “Edit…”.
- For Newer Windows 10:
You shall see a TABLE listing all the existing PATH entries (if not, goto next step). Click “New” ⇒ Click “Browse” and navigate to your JDK’s “bin
” directory, i.e., “c:\Program Files\Java\jdk-13.0.{x}\bin
“, where{x}
is your installation update number ⇒ Select “Move Up” to move this entry all the way to the TOP. - For Older Windows 10 (Time to change your computer!):
(CAUTION: Read this paragraph 3 times before doing this step! Don’t push “Apply” or “OK” until you are 101% sure. There is no UNDO!!!)
(To be SAFE, copy the content of the “Variable value” to Notepad before changing it!!!)
In “Variable value” field, APPEND “c:\Program Files\Java\jdk-13.0.{x}\bin
” (where{x}
is your installation update number) IN FRONT of all the existing directories, followed by a semi-colon (;
) to separate the JDK’s bin directory from the rest of the existing directories. DO NOT DELETE any existing entries; otherwise, some existing applications may not run.Variable name : PATH Variable value : c:\Program Files\Java\jdk-13.0.{x}\bin;[do not delete exiting entries…]
Note: If you have started CMD, you need to re-start for the new environment settings to take effect.
Step 4: Verify the JDK Installation
Launch a CMD
via one of the following means:
- Click “Search” button ⇒ Type “cmd” ⇒ Choose “Command Prompt”, or
- Right-click “Start” button ⇒ run… ⇒ enter “cmd”, or
- Click “Start” button ⇒ Windows System ⇒ Command Prompt
Issue the following commands to verify your JDK installation:
- Issue “
path
” command to list the contents of thePATH
environment variable. Check to make sure that your JDK’s “bin
” is listed in thePATH
.path PATH=c:\Program Files\Java\jdk-13.0.{x}\bin;other entries… - Issue the following commands to verify that JDK/JRE are properly installed and display their version:// Display the JDK version javac -version javac 13.0.1 // Display the JRE version java -version java version “13.0.1” 2019-10-15 Java(TM) SE Runtime Environment (build 13.0.1+9) Java HotSpot(TM) 64-Bit Server VM (build 13.0.1+9, mixed mode, sharing)
Step 5: Write a Hello-World Java Program
- Create a directory to keep your works, e.g., “
d:\myProject
” or “c:\myProject
“. Do NOT save your works in “Desktop” or “Documents” as they are hard to locate. The directory name shall not contain blank or special characters. Use meaningful but short name as it is easier to type. - Launch a programming text editor (such as TextPad, NotePad++, Sublime Text, Atom). Begin with a new file and enter the following source code. Save the file as “
Hello.java
“, under your work directory (e.g.,d:\myProject
)./* * First Java program to say Hello */ public class Hello { // Save as “Hello.java” under “d:\myProject” public static void main(String[] args) { System.out.println(“Hello, world!”); } }
Step 6: Compile and Run the Hello-World Java Program
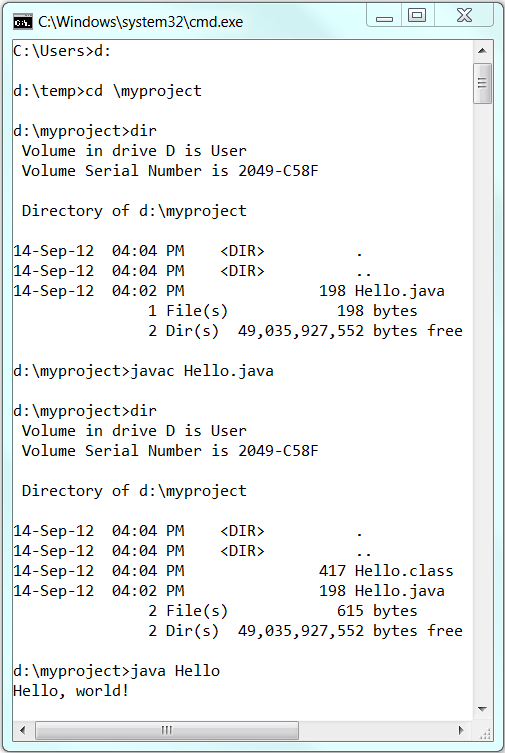
To compile the source code “Hello.java
“:
- Start a CMD Shell (Search ⇒ enter “cmd” ⇒ select “Command Prompt”).
- Set the Current Drive to the drive where you saved your source file “
Hello.java
“.
If you use drive “c”, skip this step.
Else if you use drive “d
“, enter “d:
” as follow:d: D:\xxx> - Set the Current Working Directory to the directory that you saved your source file via the
cd
(Change Directory) command. For example, suppose that your source file is saved in directory “myProject
“.
cd \myProject D:\myProject> - Issue a
dir
(List Directory) command to confirm that your source file is present in the current directory.dir …… xx-xxx-xx xx:xx PM 277 Hello.java …… - Invoke the JDK compiler “
javac
” to compile the source code “Hello.java
“.javac Hello.java // If error message appears, correct your source code and re-compileThe compilation is successful if the command prompt returns. Otherwise, error messages would be shown. Correct the errors in your source file and re-compile. Check “Common JDK Installation Errors“, if you encounter problem compiling your program. - The output of the compilation is a Java class called “
Hello.class
“. Issue adir
(List Directory) command again to check for the output.dir …… xx-xxx-xx xx:xx PM 416 Hello.class xx-xxx-xx xx:xx PM 277 Hello.java ……
To run the program, invoke the Java Runtime “java
“:
java Hello Hello, world!
Everything that can possibly go wrong will go wrong: Read “JDK Installation Common Errors“.
Step 7: (For Advanced Users Only) JDK’s Source Code
Source code for JDK is provided and kept in “<JAVA_HOME>\lib\src.zip
” (or “<JAVA_HOME>\src.zip
” prior to JDK 9). I strongly recommend that you to go through some of the source files such as “String.java
“, “Math.java
“, and “Integer.java
“, under “java\lang
“, to learn how experts program.